Send alerts to multiple platforms from your scripts
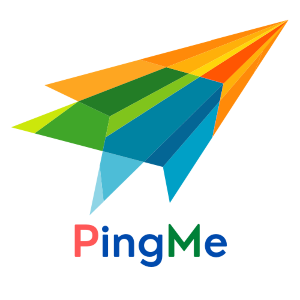
In this article, we will illustrate how to configure and send messages to multiple platforms from your scripts. A notification can be setup based on success or failure of a task or if you want to have an alert when a certain condition is meet. This article uses a small binary PingMe to send this notification, you can find more information about it on github.
Lets dig right into it
In this first example we would like to get a notification when any one logs into our server via ssh. Multiple options are available for messaging platform in this example we will use Pushover service.
- Download & install
First step is to download install the binary itself on the system which we will use for sending notifications. We can download the binary from github and extract in the current folder.
Its executable but for good measure we will make it executable and then move the binary in system path, in this case its /usr/local/bin
.
App is also available as deb and rpm, for windows users a compatible binary is can be found under release page.
1wget -q https://github.com/kha7iq/pingme/releases/download/v0.1.5/pingme_Linux_x86_64.tar.gz && tar -xf pingme_Linux_x86_64.tar.gz
2chmod +x pingme
3sudo mv pingme /usr/local/bin/pingme
Lets create our script and configure notifications
Send Alert when any user login via ssh
/etc/profile.d/
is read at every login (for bash shell users). The if
statement will only return true
if the user has logged in via ssh, which in turn will cause the indented code block to be run.
Create a file with desired name i.e /etc/profile.d/login.sh
and paste the following.
1pushover_token="<your token here>"
2pushover_user="<your user here>"
3alert_title="Alert SSH Login: $(date)"
4if [ -n "$SSH_CLIENT" ]; then
5 PUSHOVER_MESSAGE="ssh login to ${USER}@$(hostname -f) from $(echo $SSH_CLIENT|awk '{print $1}')"
6 pingme pushover --msg "$PUSHOVER_MESSAGE" --token $pushover_token --user $pushover_user --title "$alert_title"
7fi
Pushover Alert
Send alert on failure or success of a cronjob
We have a cronjob which runs and take backup of our files, we would like to know if this backup has been created successfully or failed. In this example we are going to send the alert to telegram channel.
- Cron Job
1* * * * * /home/user/scripts/backup.sh
- Backup Script
1#!/bin/bash
2# telegram bot token
3token="<token here>"
4# Telegram channel
5channel="<channel here>"
6DATE=$(date +%d-%m-%Y-%T)
7BACKUP_FILE_NAME="postgres_db-$DATE.tar.gz"
8BACKUP_DIR="/home/user/backup"
9
10# take each backup with separate name, use below format
11tar -zcpf $BACKUP_DIR/$BACKUP_FILE_NAME $HOME/dbfiles
12
13# check the exit code and send alert based on that.
14if [ $? -eq 0 ]
15then
16 title="Success: Backup created successfully"
17 /usr/local/bin/pingme telegram --msg "Successfully backed up $BACKUP_FILE_NAME" -t $token -c $channel --title "$title"
18else
19 title="Failed: Error creating backup"
20 /usr/local/bin/pingme telegram --msg "Unsuccessful backup of $BACKUP_FILE_NAME" -t $token -c $channel --title "$title"
21fi
Telegram Alert